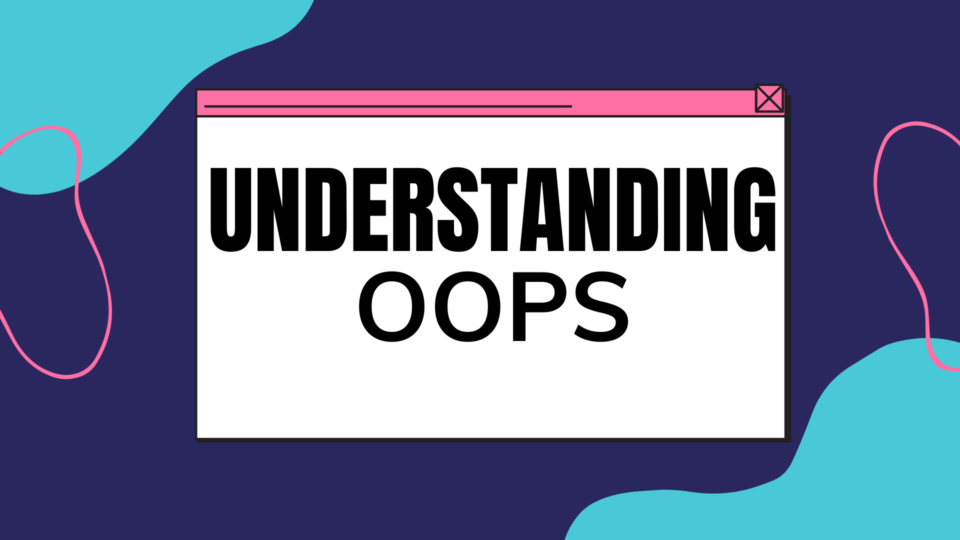
Unlocking OOP’s Secrets: A Complete Beginner’s Guide
Programming novices may be familiar with “Object-Oriented Programming” (OOP). Why is OOP so popular, and what is it? This comprehensive beginner’s book will reveal OOP’s secrets, benefits, and implementation in many programming languages.
Knowing OOP Principles
Object-Oriented Programming divides code into objects, which are class instances. This method builds software by modeling real-world elements and interactions. OOP emphasizes encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation bundles data and operations within a class to hide data and provide a controlled interface to the object. Classes can inherit attributes and methods from other classes, allowing code reuse and hierarchical relationships. Polymorphism makes items flexible and extensible by changing their appearance and behavior. Complex systems are simplified by abstracting important characteristics and hiding superfluous aspects.
Benefits of OOP
OOP has several benefits for programming. Modularity lets you split large systems into smaller, manageable bits. This modular method organizes, reuses, and simplifies code maintenance and updates. OOP saves development time by reusing code through inheritance.
Other benefits of OOP include precise modeling of real-world entities. Using objects and classes, you can construct software that behaves like real objects. This simplifies, clarifies, and reduces errors in the code. OOP also provides encapsulation, which protects sensitive data from unauthorized access.
Key OOP Concepts: Classes and Objects
Classes and objects are OOP’s software building pieces. Class templates define object properties and actions. It contains entity-specific data and methods. However, objects are class instances. It represents a class entity instance.
For example, imagine the “Car.” class. A Car class would define color, brand, model, and methods like start(), stop(), and accelerate(). Each object created from this class has its own property values and can call class methods.
class Car:
def __init__(self, color, brand, model):
self.color = color
self.brand = brand
self.model = model
def start(self):
# Code to start the car
def stop(self):
# Code to stop the car
def accelerate(self):
# Code to accelerate the car
Classes and objects allow you to write modular, reusable code that appropriately models entities and actions.
Data Hiding and Encapsulation in OOP
OOP’s encapsulation principle hides data and controls object access. It lets you bundle data and operations within a class to safeguard sensitive data and provide a clear object interface.
Encapsulating data prevents unwanted access and change, protecting code integrity. Consider a bank account class. The account balance should not be visible or changeable outside the class. Instead, define deposit() and withdraw() to manipulate the balance internally.
class BankAccount:
def __init__(self):
self.balance = 0
def deposit(self, amount):
# Code to add amount to balance
def withdraw(self, amount):
# Code to subtract amount from balance
Encapsulating the balance characteristic and offering regulated methods to interact with it enforces business rules and prevents unintentional changes, protecting the bank account.
Polymorphism and Inheritance in OOP
Strong OOP functionality called class inheritance allows classes to take methods and properties from other classes. This encourages code repetition and class hierarchies.
In OOP, a subclass or derived class inherits from a superclass or base class. All superclass characteristics and behaviors are passed to the subclass, which can modify them.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError(“Subclass must implement this method”)
class Dog(Animal):
def speak(self):
return “Woof!”
class Cat(Animal):
def speak(self):
return “Meow!”
Animal is the superclass, while Dog and Cat are subclasses. Their name attribute comes from Animal, and they implement talk(). This lets us construct Dog and Cat objects and call speak() to receive distinct outcomes.
OOP’s polymorphism lets objects of different classes behave like those of a superclass. This means that a superclass variable can hold objects of any subclass, and the method implementation will be invoked based on the object type.
def make_animal_speak(animal):
print(animal.speak())
dog = Dog(“Buddy”)
cat = Cat(“Whiskers”)
make_animal_speak(dog) # Output: Woof!
make_animal_speak(cat) # Output: Meow!
Inheritance and polymorphism allow you to write versatile, extendable code that handles several object types.
Interfaces and Abstraction in OOP
Abstraction in OOP simplifies complex systems by representing important aspects and hiding superfluous details. It lets you focus on object and class functionality rather than implementation.
Interfaces can abstract. A class must implement a contract of methods defined by an interface. It guides the creation of classes with identical behavior but diverse internal implementation.
from abc import ABC, through abstractmethod
class Shape(ABC):
@abstractmethod
def calculate_area(self):
pass
class Rectangle(Shape):
def __init__(self, length, width):
self.length = length
self.width = width
def calculate_area(self):
return self.length * self.width
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def calculate_area(self):
return 3.14 * self.radius ** 2
For instance, the Shape class interface defines calculate_area(). Rectangle and Circle classes use this method for their calculations. By using the Shape interface, you may develop code that works on any object that supports it without worrying about its internals.
Abstraction and interfaces make code modular, versatile, and manageable. They segregate implementation details from high-level functionality, making code modifications and extensions easy without harming other areas of the system.
OOP vs. Procedural
Before diving into OOP, we must distinguish it from procedural programming. Traditional procedural programming involves writing data-operating procedures or functions. To complete a task, the main program calls various functions sequentially.
In contrast, OOP arranges code into objects that contain data and methods. It models real-world entities and their interactions to make the issue domain more comprehensible. Polymorphism and these things makes OOP programming easy to modify, extend, and reuse.
OOP is better for large, complicated projects that need code organization and maintainability. Procedural programming may be better for simple jobs that don’t require real-world entity modeling.
Different Programming Languages for OOP
No programming language is required for OOP. The programming paradigm can be used in Python, Java, C++, and others. While each language has its own syntax and norms for working with objects and classes, OOP fundamentals remain the same.
Let’s briefly examine Python’s OOP implementation, a popular introductory programming language:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f”Hello, my name is {self.name} and I’m {self.age} years old.”)
person = Person(“John”, 25)
person.greet() # Output: Hello, my name is John and I’m 25 years old.
This sample creates a Person class with greet(), age, and name. To send a personalized greeting, we construct a Person object and use greet().
The essential principles and concepts of OOP are identical across computer languages, however implementation may vary. Understanding OOP basics lets you apply them to any language.
Top OOP Programming Tips
To write clean, maintainable, and efficient OOP code, follow best practices. Consider these guidelines:
1. Minimize and prioritize classes:
Each class should have one responsibility to simplify understanding, testing, and maintenance.
2. Name things properly:
To improve code readability, name classes, methods, and variables descriptively.
3. Avoid excessive inheritance:
While inheritance is powerful, overuse can lead to complex, tightly-coupled programs. Use inheritance only for “is-a” relationships.
4. Prefer composition over inheritance:
Compose objects of other classes within your class instead of inheriting from numerous classes to integrate their behaviors.
5. Design for extensibility:
Plan for future modifications and make your classes and interfaces flexible without rewriting code.
6. Apply the least privilege principle:
To maintain encapsulation and data integrity, restrict attribute and method access.
These best practices help you build clear, maintainable, and robust OOP code.
Design patterns for OOP
Common software development design difficulties are solved by design patterns. They offer proven solutions to problems and encourage code reuse, maintainability, and extensibility.
OOP-specific design patterns include Singleton, Factory, Observer, and Strategy. Patterns solve specific problems with standardized solutions that may be applied to numerous situations.
This beginner’s guide doesn’t cover each design pattern, but it’s important to know about them. You may write more efficient and adaptable OOP programs by understanding design patterns.
Common OOP Misconceptions
As with any complicated topic, OOP has widespread misconceptions that might confuse. Some myths are clarified:
7. OOP is the only method to build good code:
While OOP is strong, it is not the only way to write effective code. Functional programming has its own strengths and may be better for some situations.
8. OOP only for big projects:
OOP works best in large projects but can benefit smaller ones. The modular and reusable structure of OOP improves code organization and maintainability in any project size.
9. OOP is too difficult for beginners:
OOP is complicated, but beginners may understand and use its fundamentals. Beginners can appreciate OOP by starting with simple examples and developing on them.
10. OOP replaces procedural programming:
OOP often uses procedural programming despite its concentration on objects and classes. OOP languages often offer procedural programming in the same codebase.
11. OOP consume more energy:
It’s true OOP consumes little more energy. Not only OOPs, every programming language which has a long code to process eventually consumes more energy. To learn more about energy consumption and crypto related things visit alertacripto.
Understanding these myths can help you use OOP wisely and recognize its drawbacks.
OOP Learning Resources
There are several resources to help you learn and master OOP. Some suggested resources:
- Books: “Clean Code” by Robert C. Martin.
- Online courses: Udemy offers several excellent OOP courses.
- Coding exercises and challenges: You can practice coding on Leetcode and Hackerrank.
Continued practice and real-world tasks will help you master OOP.
Conclusion:
This comprehensive beginner’s course explains OOP and its benefits. OOP simplifies complex systems, increases code reuse, and enhances maintainability through objects, encapsulation, inheritance, polymorphism, and abstraction. For programmers of all skill levels to develop readable, effective, and flexible code, they must grasp OOP. Try OOP, use best practices, play with design patterns, and never forget that OOP may help projects of any size. Enjoy your coding!